The difference between a method and a function - Coding Basics
Some OOP for you
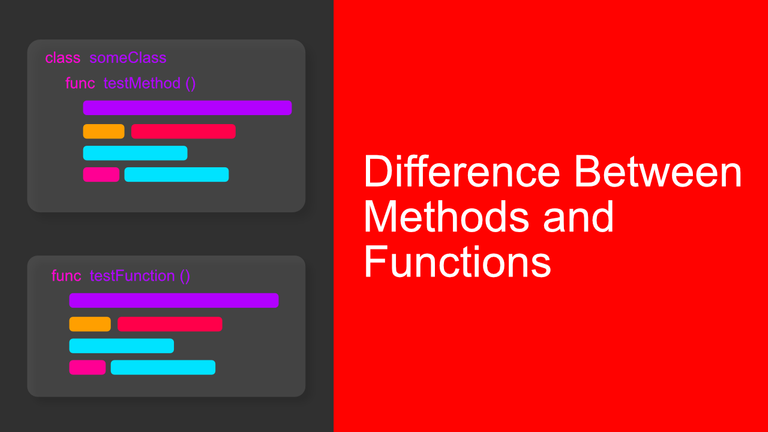
Shoutout to Programming with Swift
In this article you'll find:
- Introduction
- What is a function?
- What is a method?
- So, what are the differences?
One of the main unknowns for those starting in the world of programming is the difference between a function and a method. While the way they operate may seem similar, they differ in certain characteristics, which will be key to understanding how each works.
That is why, in the following article I will explain the differences between methods and functions, so that you have total clarity when facing projects that have these two types of instructions.
What is a function?
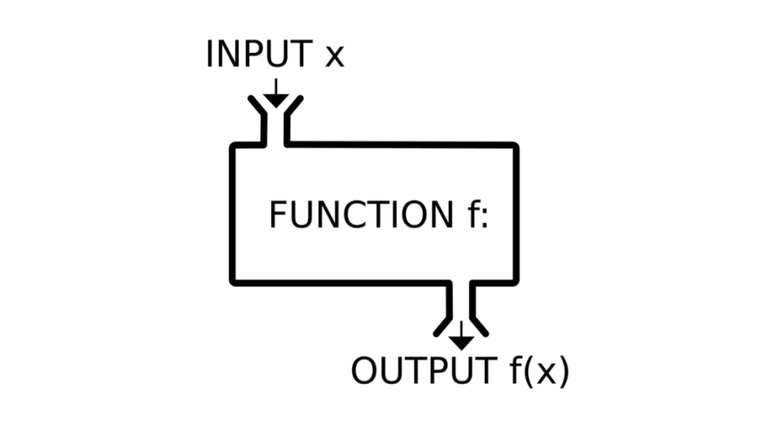
Shoutout to The Tech Edvocate
If you look at the code of any program, you will most likely come across lines of code like the following:
def create_app():
app = Flask(__name__)
app.config.from_mapping(
DEBUG = True,
SECRET_KEY = 'dev',
SQLALCHEMY_DATABASE_URI = "sqlite:///project.db"
)
db.init_app(app)
from . import todo
app.register_blueprint(todo.bp)
from . import auth
app.register_blueprint(auth.bp)
@app.route('/')
def index():
return render_template('index.html')
with app.app_context():
db.create_all()
return app
If you find this, congratulations, you've just seen a function. A function, routine, subprogram, subroutine, or procedure is a set of instructions that carry out a task (they can perform more than one, although it is not recommended), all packed in a single unit that we can execute later in the program.
If we wanted the previous function to be executed, we would only have to type:
create_app()
And it would execute our function at that instant. However, not all functions will have this form. Sometimes, we will have to work with arguments, but what is an argument?
Similar to a mathematical function, an argument is an input variable or value that we place in our function to give us a result using it.
An easier way to explain this is by defining a function that adds two numbers together. This would look like this:
def sum_two_num(a,b):
return a + b
If we take a look at the "a,b" section inside the parentheses, those will be our arguments. These will act inside the function, but nothing will happen if we don't give them a value when we call it. If we use:
print(sum_two_num(2,4))
This will give us the result 6. If we want to add two other values, we only need to change the numbers. Not only this, but we can also enter variable names, but we must be careful with the datatype.
What is a method?
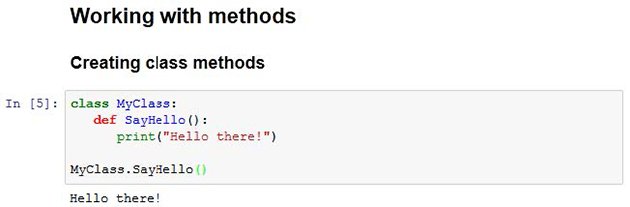
Shoutout to Dummies.com
A method, like a function, is a set of instructions that execute a task, but the main difference is that they will always be associated to an object, that is, the instance of a class.
If we take a look at the following code:
var str = 'Changethis';
var str1 = str.toLowerCase();
var str2 = str.toUpperCase();
We will see that str1 and str2 execute methods (toLowerCase(), toUpperCase()), which are not called by themselves. Since they are associated with str (the object), they will execute the instructions to place the uppercase or lowercase characters on str. If we print str1 and str2, we will have:
* str1: changethis
* str2: CHANGETHIS
And just as in a function, parameters can be added to these if the method requires it. Take a look at the following one:
learning = ['python', 'javascript', 'C#']
print(learning.index('javascript'))
We see how the 'javascript' parameter will be used to look up the index of the string javascript, which is the second item in our learning list, which will be our object. When we execute the print, this will return 1, since we start counting from 0 in programming.
However, so far we have only taken a look at built-in class methods in Python. These classes are those that are already defined as part of Python and that we can refer to whenever we want to use them.
If we decided to create our own class and our own methods, there would be no problem. If we take a look at the following code, we can create our own class and methods.
class User():
id = 2
def name(nam):
return(f "Hello, {nam}").
name1 = User.name("John")
print(name1)
We will see that the User class was created, containing an id attribute and even an internal function (Our method), which takes nam as an argument and returns a greeting.
If we call the method with the argument John, we would get "Hello, John", which confirms that a method is nothing more than a function that refers to an object.
So, what are the differences?
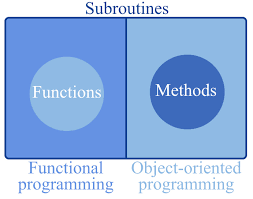
Shoutout to Baeldung
To conclude, the main differences that we can notice between methods and functions are:
Functions are a set of instructions that can be called independently, while a method must always be called by its object.
A function operates with the arguments we assign to it and with its internal variables, while a method can work with all the data inside the class of the object it accompanies.
Thus, we can see the differences between these two.
The world of programming is really extensive, and with this, information tends to get confused, especially similar concepts. However, with this small guide, you will be able to easily distinguish a function from a method. If you would like to see more posts like this one, comment what you would like to know.
Thank you for your support and good luck!
Congratulations @jesalmofficial! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 200 comments.
Your next target is to reach 200 replies.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Check out our last posts:
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.