Python Loops and Tricks By albro
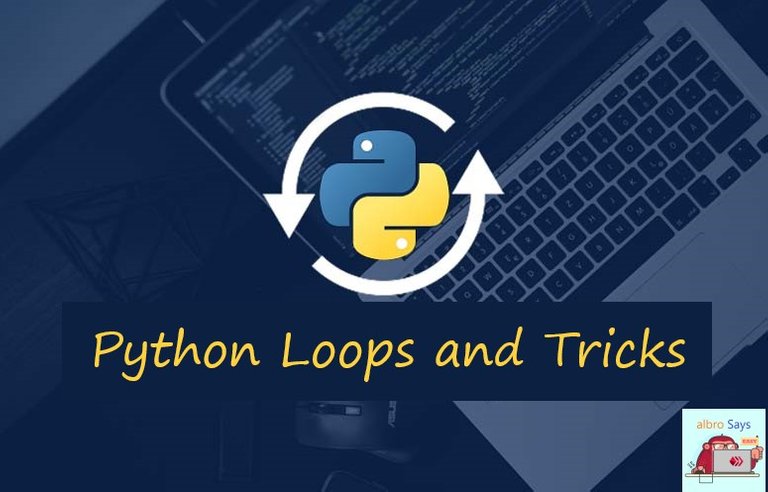
With the help of loops, which are part of control statements, we can navigate through sequences or repeat a piece of code several times. In this post, I'll talk about loops in Python and we will get to know the 2 main types and tricks to work with them.
Suppose you have several numbers in a Python list that you want to add together. Or you have a text string that you want to check character by character. Perhaps you want the Python program to perform a static or similar task as long as a condition is met.
You can use Python Loops to do all these things. Loops are one of the most used control statements.
Types of loops in Python
In the Python programming language, we have two main types of loops:
while
loopfor
loop
Each of these loops is used in its own place, but the main work of both is almost the same.
If you get a little creative, you can probably convert these two types of loops and use them interchangeably! But it is better to use each one in its proper place.
The following image shows a general flowchart (or process) of the function of loops in programming.
Sometimes it's necessary to initialize one or more required variables at the beginning of the work. Then we define a condition for the loop.
As long as that condition is met, the piece of code will be executed within the scope of the loop. After each code execution, the desired condition is checked again. Whenever the condition is not met, the loop will be exited and the program will continue.
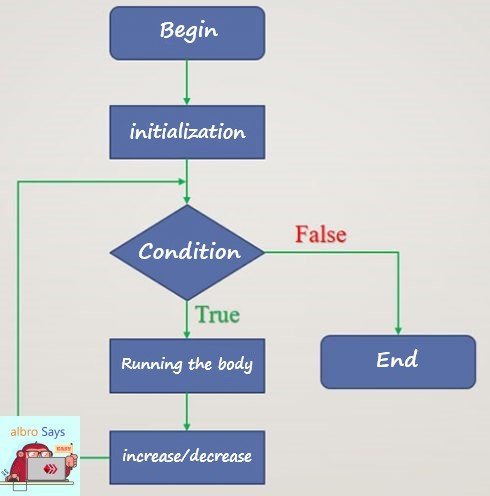
While loop in Python
By using the while
loop, a set of codes can be executed until the desired condition is met.
The structure (syntax) of the while
loop in Python is as follows:
while condition:
#code
condition
is our condition whose output must be True
or False
.
Suppose we have a variable called i
. We want to print the value as long as it's less than 5. Pay attention to the following code snippet:
i = 1
while i<5:
print(i)
i+=1
We defined the condition of the while
loop as i<5
. In the body of the loop, we have printed the value of the variable and finally increased its value by one unit.
If we don't increase the value of the variable in the last line, our loop will run infinite.
In such cases, it's usually said that we have encountered an Infinite loop!
The executed output of this piece of code will be as follows:
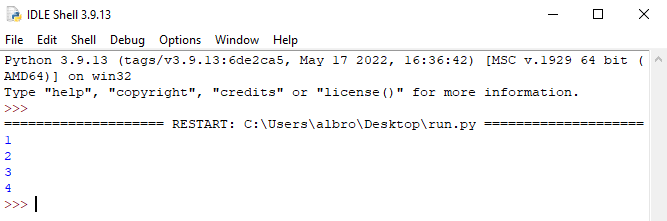
For loop in Python
The for
loop, which is also known as the for in
loop in Python, is mostly used for traversal.
Suppose you have a tuple in python. This tuple gives us a sort of sequence of its members. If we want to move on each of these elements, we will use the for
loop.
In general, anything that gives us a sequence of elements (collection) we can put in a Python for
loop; Data types list, string, tuple, etc.
The general structure of the for
loop in Python is as follows:
for Value in Sequence:
#execution and use of Value
The for
loop syntax has three main parts:
Value
: the name of the variable in which each element of the collection is placed each time the loop is executed.Sequence
: The scrollable collection we want.Loop Body
: The code that is processed each time the loop executes.
Suppose we have a list that contains the names of five Hive users. Using the following code, we perform the traversal operation in the list:
names = ["albro", "ecency", "peakd", "leofinance", "hive"]
for name in names:
print("Hello " + name + "!")
The output of this loop will be as follows:
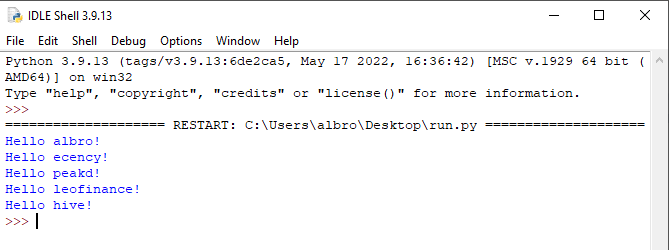
String in Python is also a collection; A set of characters that make up a text string. Therefore, with the help of the for in
loop, it is possible to traverse through each character of a string.
string = "Welcome to albro Hive blog!"
i = 0
for char in string:
i+=1
print("Lenght is: " + str(i))
In the code above, we calculated the number of characters in the string using the for
loop.
With the help of iterators in Python, you can create a traversable object in python loops as you like.
range
function
Suppose we want to create a for
loop whose variable increases by one each time it is executed. Something similar to what we had in the while
loop.
Suppose we want to calculate the sum of the numbers 1 to 9 with a loop.
The first solution is to manually create a list of consecutive numbers and use it in a for
loop.
nums= [1, 2, 3, 4, 5, 6, 7, 8, 9]
result = 0
for n in nums:
result += n
print(result)
But we have a simpler solution!
The range()
function creates a list of integers. This function can be used directly in a for
loop in Python to generate a range of numbers (numeric range).
The following code is exactly equivalent to the above code and gives us the number 45 as a result.
result = 0
nums = range(10)
for i in nums:
result += i
print(result)
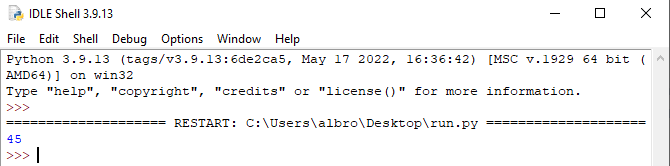
The range(n)
function gives us a list of numbers from 0
to n-1
.
This function can be used in a more professional way. Its general structure is as follows:
range(start, stop, step)
The three input arguments of this function are:
start
: the starting point of countingstop
: end point (this point won't be part of the numerical sequence)step
: the step of the movement from the beginning to the end (optional, the default number is considered 1)
If only one input is entered for range()
, by default the start value will be 0 and the step will be 1.
Consider the following examples of this function:
range(9)
# [0, 1, 2, 3, 4, 5, 6, 7, 8]
range(5, 21)
# [5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
range(5, 21, 3)
# [5, 8, 11, 14, 17, 20]
Tricks of working with loops
There are many tricks to working with loops in Python. Most of these tricks are due to the programmer's creativity during programming.
In order to be able to program more professionally with loops, there are two important statements and structures.
In the following, I'll check the break
and continue
statements in loops and Nested Loops structure.
The break
statement in a Python loop
Suppose we have a list of numbers and we want to traverse it. If we reach a certain number, the entire collection will end.
What happens after we reach the desired number?! We must completely exit the loop (for
or while
)!
To exit the loop, we use the break
statement.
As soon as the break
statement is reached, it will be immediately exited from the loop and subsequent repetitions will be ignored; Regardless of whether we are in the first iteration of the loop or the last one!
Usually, this kind of work is done with the help of condition structures in Python.
lst = [1, 2, 3, 4, 5, 6, 7, 8, 9]
for i in lst:
if i == 5:
break
print(i)
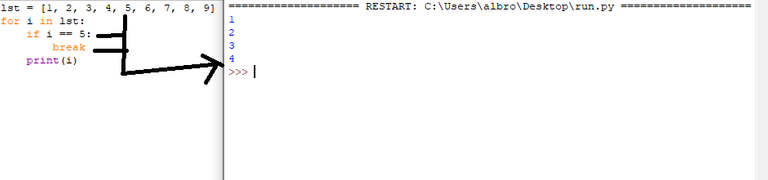
The output of this piece of code is only the numbers 1 to 4.
Using the continue
statement
In the same previous example, what should be done if we just want a certain number not to be printed?
The first solution is probably to use a condition. so that if the selected element isn't equal to 5, the print
statement will be executed.
Another solution is to use the continue
statement. By executing continue
in the loop, the rest of the loop body code isn't executed and we jump directly to the beginning of the next iteration in the loop.
Pay attention to the following code:
lst = [1, 2, 3, 4, 5, 6, 7, 8, 9]
for i in lst:
if i == 5:
continue
print(i)
The result of executing this code will be as follows:
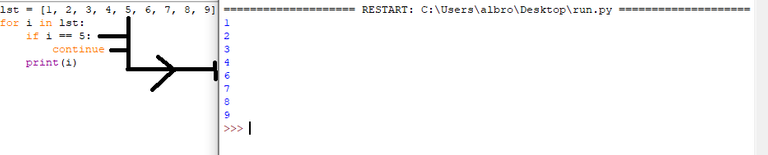
As you can see, except for the number 5, the rest of the numbers in the list are printed in the output.
Nested Loop in Python
Python Loops can also be used in the form of nest. For example, the matrix can be traversed as follows. Note that the range of the loop body codes in the Python is determined by the same indentation
.
mtrx = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
x = 0
for i in mtrx:
for j in i:
x += j
print(x) #45

Summary: Loops in Python
In this post we are introduced to how to define the loop in Python. One loop has two main parts of the condition and the body. Before the condition, we can do it for that initialization. Then, as long as the condition is in place, the codes are executed inside the loop body. In order not to face the infinite loop problem, it is best to consider how the condition is changed. Increased operations are usually used to do this.
In each of the for
Python loops, the variable we have defined takes a new amount and the body codes are implemented. Note that we can use this variable in the body or not! The While loop is executed in Python as long as the condition is true.
In addition to the loops, we introduced the range()
function to generate a list of numbers, the break
statement to stop running the loop and the continue
statement to jump to the next loop.
https://leofinance.io/threads/view/albro/re-leothreads-2j4swbfgj
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 3500 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out our last posts:
Support the HiveBuzz project. Vote for our proposal!
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🏅 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.